- Published on
Python tip N2 : function call overhead
- Authors
- Name
- Ismail Tlemcani
- @Ismailtlem
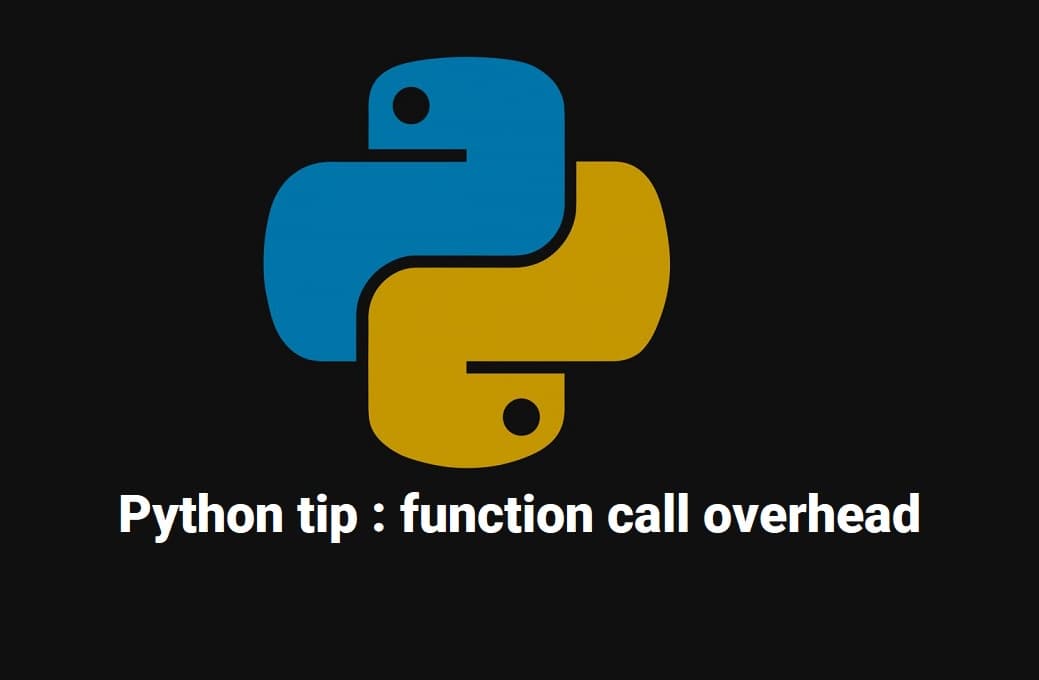
In this article, we'll see a python performance tip to avoid in your code. I first came across this tip while watching this talk on Python performance
Situation ?
You have a hot loop in your code (a loop iterating over many items like the one below, and which can have a serious effect on the program's performance). Should you call a function inside this loop ? Better no. It's also what Guido van Rossum has suggested in this post for writing faster Python
for number in range(1_000_000):
Solution
Instead of calling the separate function, consider performing the operations directly within your loop. It's for sure not very Pythonic and it's more code, but it's better from a performance point of view
Example
You can test this tip from the following example
import time
def multiply(x,y):
return x*y
def a():
start = time.time()
x = 1
for number in range(1_000_000):
multiply(x,number)
print(time.time() - start)
def b():
start = time.time()
x=1
for number in range(1_000_000):
x*number
print(time.time() - start)
On my local setup, running the function a gives the following result
0.06376457214355469
and running the function b gives the following result
0.030170440673828125
So it's a 48 % performance improvement
Why is that ?
In a nutshell, a function call requires that a new stack frame is created and pushed into the stack and that operation is expensive. In addition to that, the python interpreter does no optimization to your code and runs it as it is. You can watch this talk for more details on how Python implements stack frames.
Happy Coding !